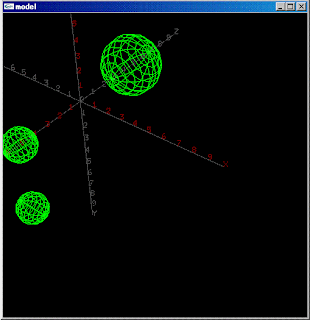
# u(+y) i(-z)
# h(-x) j(reset) k(+x)
# n(+z) m(-y)
レンズの光軸の指す点を3次元座標で指定するのですが、たとえばキー"k"を押すと、その座標が+1されて、カメラがちょっと右向きます。すると景色は左に動きます。
I can pan the camera by using gluLookAt() command and keyboard. Push a key, u, i, h,j,k,n, or m, camera will pan accordingly.
# u(+y) i(-z)
# h(-x) j(reset) k(+x)
# n(+z) m(-y)
For example, push 'k' key once, x=x+1. You pan right your camera to a point x+1, y, z. Thus, objects you are seeing will move to left.
import sys
from OpenGL.GL import *
from OpenGL.GLU import *
from OpenGL.GLUT import *
# draw x, y, z axis with index
# draw 3 sphere
# use keyboard to pan camera from a position (20,20,20)
#
# u(+y) i(-z)
# h(-x) j(reset) k(+x)
# n(+z) m(-y)
def display():
glClear(GL_COLOR_BUFFER_BIT)
axis()
glColor3f(0.0, 1.0, 0.0)
mySphere = gluNewQuadric()
gluQuadricDrawStyle(mySphere, GLU_LINE)
glTranslatef(0.0, 0.0, -5.0)
gluSphere(mySphere, 2.0, 12, 12)
glTranslatef(0.0, 0.0, 10.0)
gluSphere(mySphere, 1.0, 12, 12)
glTranslatef(0.0, -5.0, 0.0)
glRotatef(90.0, 0.0, 1.0, 0.0)
gluSphere(mySphere, 1.0, 12, 12)
glFlush()
def axis():
glClear( GL_COLOR_BUFFER_BIT )
glBegin(GL_LINES)
glColor3f(0.3, 0.3, 0.3)
glVertex3f(-10.0, 0.0, 0.0)
glVertex3f(10.0, 0.0, 0.0)
glVertex3f(0.0, -10.0, 0.0)
glVertex3f(0.0, 10.0, 0.0)
glVertex3f(0.0, 0.0, -10.0)
glVertex3f(0.0, 0.0, 10.0)
glEnd()
biggest = 10
x = 88
y = 89
z = 90
r = range (0,(biggest+1))
for i in r:
ascii = 48+i
# x axis positive
glRasterPos3f(i, 0.0, 0.0)
glColor3f(0.3, 0.3, 0.3)
if i==biggest:
glutBitmapCharacter(GLUT_BITMAP_8_BY_13, x )
else:
glutBitmapCharacter(GLUT_BITMAP_8_BY_13, ascii)
# x axis negative
glRasterPos3f(-i, 0.0, 0.0)
glColor3f(0.5, 0.0, 0.0)
if i==biggest:
glutBitmapCharacter(GLUT_BITMAP_8_BY_13, x)
else:
glutBitmapCharacter(GLUT_BITMAP_8_BY_13, ascii)
# y axis positive
glRasterPos3f(0.0, i, 0.0)
glColor3f(0.3, 0.3, 0.3)
if i==biggest:
glutBitmapCharacter(GLUT_BITMAP_8_BY_13, y)
else:
glutBitmapCharacter(GLUT_BITMAP_8_BY_13, ascii)
# x axis negative
glRasterPos3f(0.0, -i, 0.0)
glColor3f(0.5, 0.0, 0.0)
if i==biggest:
glutBitmapCharacter(GLUT_BITMAP_8_BY_13, y)
else:
glutBitmapCharacter(GLUT_BITMAP_8_BY_13, ascii)
# z axis positive
glRasterPos3f(0.0, 0.0, i)
glColor3f(0.3, 0.3, 0.3)
if i==biggest:
glutBitmapCharacter(GLUT_BITMAP_8_BY_13, z)
else:
glutBitmapCharacter(GLUT_BITMAP_8_BY_13, ascii)
# z axis negative
glRasterPos3f(0.0, 0.0, -i)
glColor3f(0.5, 0.0, 0.0)
if i==biggest:
glutBitmapCharacter(GLUT_BITMAP_8_BY_13, z)
else:
glutBitmapCharacter(GLUT_BITMAP_8_BY_13, ascii)
glFlush()
def init():
glClearColor(0.0, 0.0, 0.0, 0.0)
glColor3f(0.0, 0.0, 0.0)
glMatrixMode(GL_PROJECTION)
glLoadIdentity()
gluPerspective(30, 1.0, 0.0, 100.0)
glMatrixMode(GL_MODELVIEW)
glLoadIdentity()
gluLookAt(20.0, 20.0, 20.0, xx, yy, zz, 0.0, 1.0, 0.0)
def mymouse(but, stat, x, y):
if stat == GLUT_DOWN:
if but == GLUT_LEFT_BUTTON:
print x, y, "Press right button to exit"
else:
sys.exit()
def myidle():
glutPostRedisplay()
def mykey(key, x, y):
global xx, yy, zz
if key=='k': # +x
xx = xx + 1.0
print "xx=", xx
elif key=='h': # -x
xx = xx - 1.0
print "xx=", xx
elif key=='u': #+y
yy = yy + 1.0
print "yy=", yy
elif key=='m': #-y
yy = yy - 1.0
print "yy=", yy
elif key=='i': #-z
zz = zz - 1.0
print "zz=", zz
elif key=='n': #+z
zz = zz + 1.0
print "zz=", zz
elif key=='j': # center camera to origin 0,0
xx=yy=zz=0.0
print "reset x,y,z to (0,0)"
glMatrixMode(GL_MODELVIEW)
glLoadIdentity()
gluLookAt(20.0, 20.0, 20.0, xx, yy, zz, 0.0, 1.0, 0.0)
glutPostRedisplay()
glutInit( sys.argv )
glutInitDisplayMode( GLUT_SINGLE | GLUT_RGB )
glutInitWindowSize( 500, 500 )
glutInitWindowPosition(0,0)
glutCreateWindow( 'model' )
glutDisplayFunc( display )
glutMouseFunc(mymouse)
glutKeyboardFunc(mykey)
xx=yy=zz=0.0
init()
glutMainLoop()
0 件のコメント:
コメントを投稿